Biggest challenge of training AI is turning web data into LLM-ready formats like Markdown.
Not anymore.
Clean and Structured,
Every Time
Our web scraping API extracts all data inside your target URL and turns it into structured Markdown format.
On top of that, our API bypasses any WAF through rotating proxies, header and UA management, and CAPTCHA bypass - so you can power your AI apps with any and all data on the public web.
# Import necessary libraries
import requests
import urllib.parse
# Set your API token
token = "YOUR_TOKEN"
# Target URL to scrape
targetUrl = urllib.parse.quote("https://httpbin.co/")
# Select Markdown as your output and construct API request URL
url = "http://api.scrape.do/?token={}&url={}&output=markdown".format(token, targetUrl)
# Make the API request
response = requests.request("GET", url)
# Print the response in Markdown format
print(response.text)
curl --location --request GET \
'https://api.scrape.do/?token=YOUR_TOKEN&url=https://httpbin.co/&output=markdown'
// Import the Axios library
var axios = require('axios');
// Set your API token
var token = "YOUR_TOKEN";
// Target URL to scrape
var targetUrl = encodeURIComponent("https://httpbin.co/");
// Choosing Markdown as output and configuring API request
var config = {
method: 'GET',
url: `https://api.scrape.do/?token=${token}&url=${targetUrl}&output=markdown`,
headers: {}
};
// Make the API request
axios(config)
.then(function (response) {
// Print the response
console.log(response.data);
})
.catch(function (error) {
console.log(error);
});
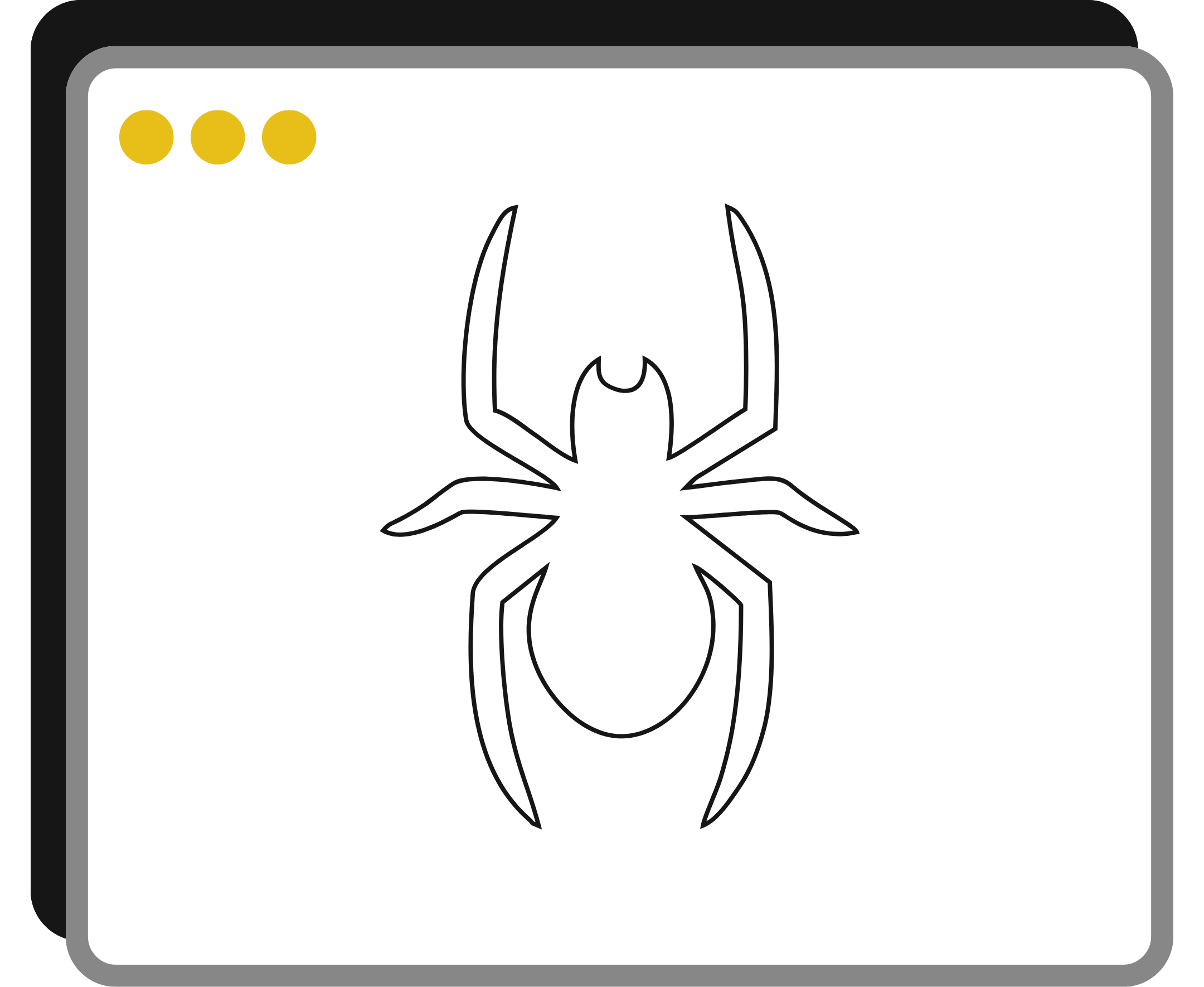
Crawl and Extract, Simultaneously
Use our open-source Python library to crawl all URLs of a website - you only need the root domain!
Our crawler uses Scrape.do to crawl and scrape at the same time so you can extract whole websites as Markdown with only a single command.
Frequently Asked Questions
LLM-ready data refers to structured, clean, and standardized content that can be directly used for training large language models (LLMs). Markdown is the ideal format because it’s lightweight, human-readable, and easy to parse. It preserves the structure of web content—such as headings, lists, and links—without unnecessary clutter, making it perfect for AI training.
With Scrape.do, you can scrape a wide range of websites, including blogs, news portals, documentation sites, and e-commerce platforms. Whether it’s static or dynamic content, our API handles it all—bypassing firewalls, managing sessions, and avoiding blocks to deliver structured data effortlessly.
Scrape.do features a built-in HTML-to-Markdown converter that automatically transforms web pages into clean Markdown format.
Scrape.do is built to scale, offering adaptive proxy rotation, header management, and CAPTCHA bypassing to ensure uninterrupted data extraction. Our robust infrastructure processes millions of requests every day with 99% success rate.
With Scrape.do, you get:
- Cost-efficient pricing: Pay only for successful requests, saving you money.
- Reliable service: 99.98% success rate and 24/7 expert support.
- Unmatched speed: 40% faster response times compared to competitors.